Introduction
Hello, this is Pike8. I have had many opportunities to write shell scripts to make my work a little more efficient, but they have often been disposable scripts or ad hoc development, and I have never set up a proper development environment. Code formatting was done by hand, nthe main way to check logic was print debugging, and I used bashdb as a debugger on the terminal.
Recently, I have been writing more and more shell scripts personally, so I set up a Shell development environment with VSCode. This time, I would like to leave a log of the work.
What I want to do
Using VSCode, I want to set up a development environment for Shell, mainly Bash.
Specifically
- Code auto completion
- Jump to code definition
- Automate code formatting
- Automation of static analysis
- Use of debuggers on the IDE
- and more
Environments
- macOS: Ventura
- VSCode: 1.78.2
Setting up the environment
Installing extensions
Search for and install the following extensions from Extensions
- Bash IDE
- shell-format
- ShellCheck
- Bash Debug (if you use a debugger)
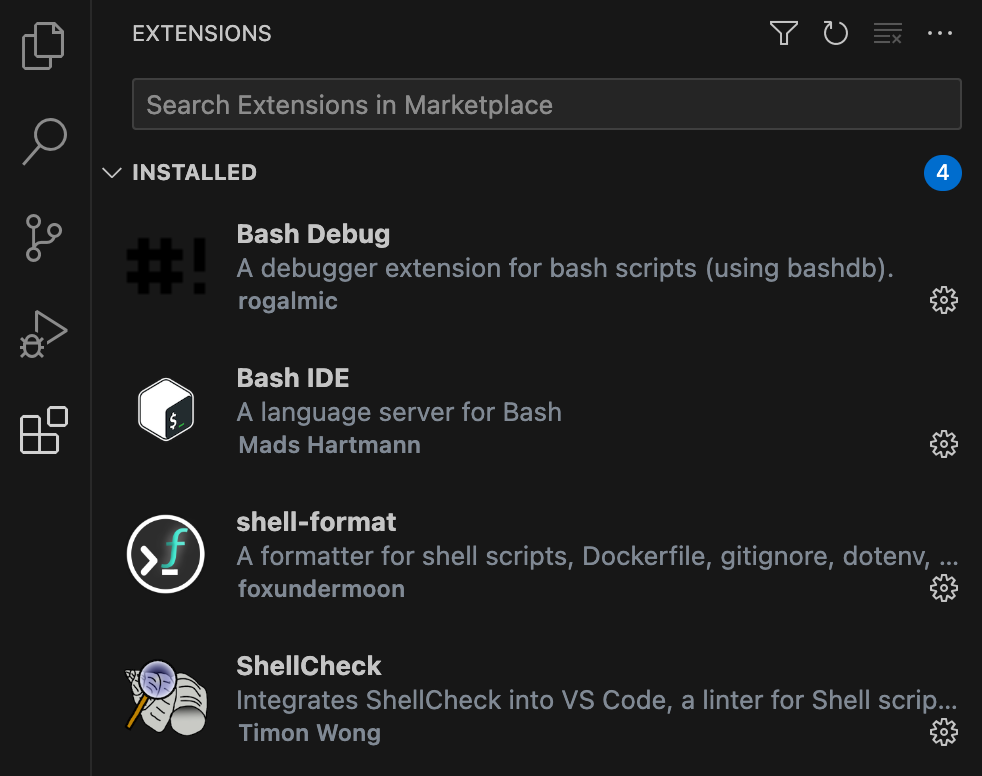
VSCode Preferences
In Settings
, check Text Editor > Formatting > Format On Save
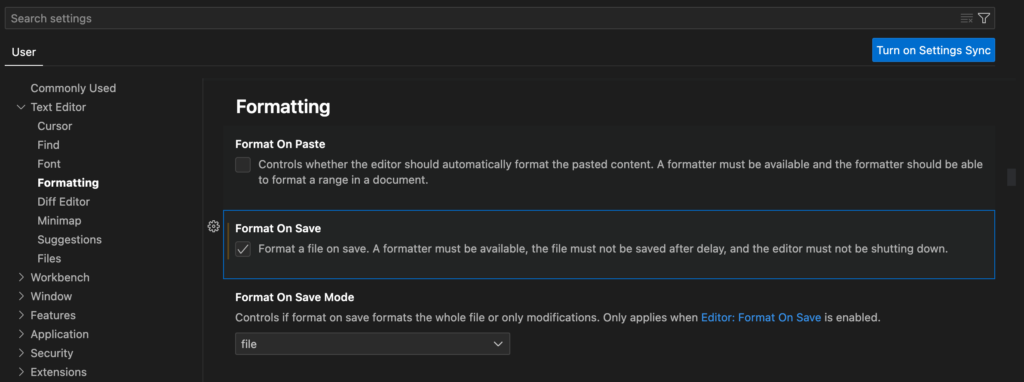
Update Bash (if using debugger)
If your Bash version is older than 4.0, you need to update it to 4.0 or higher
If Homebrew is not installed, install Homebrew with the following command in the terminal
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
Install the latest version of Bash with the following command in the terminal
brew install bash
Add Launch file (if using debugger)
Add the following to .vscode/launch.json
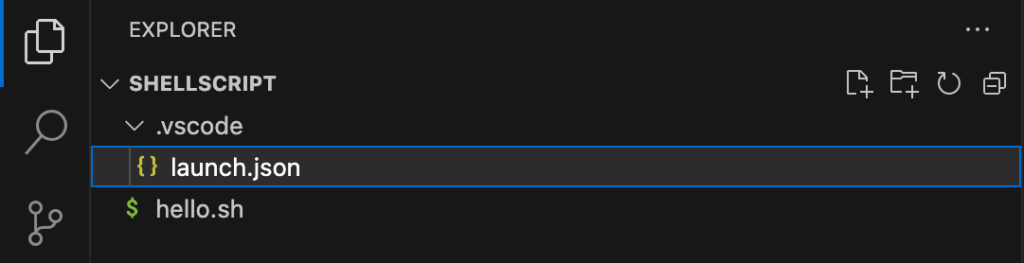
{
"version": "0.2.0",
"configurations": [
{
"type": "bashdb",
"request": "launch",
"name": "Bash Debug",
"program": "${file}",
"terminalKind": "integrated"
}
]
}
Explanation
Bash IDE
You can use the features described on this page simply by installing the extension. Some of them are listed below.
Code completion
Automatically completes commands or snippets of code that you have typed in the middle of a sentence.
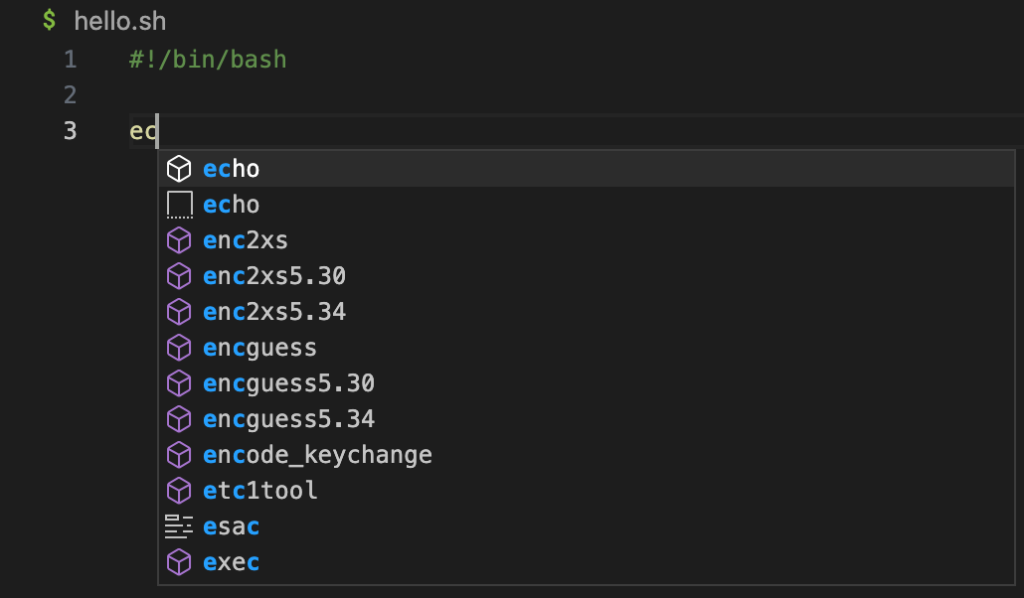
Go to definition
You can move to the definition of a function or variable by clicking on it while holding down command
, or by pressing F12
with the cursor over it. You can also use Shift + F12
to find the code you are referring to.
Viewing the Documentation
Mouse over a function or variable to display its documentation.
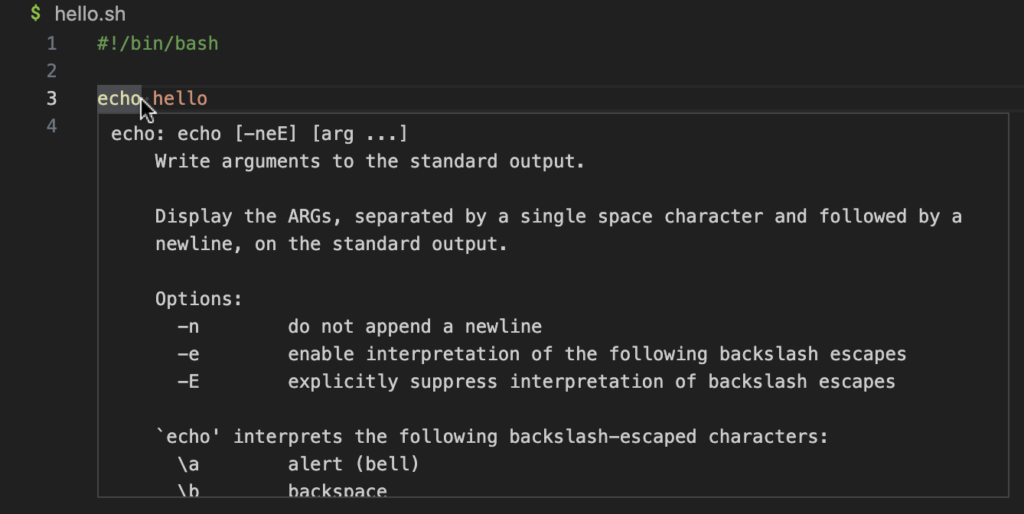
shell-format
If you install the extension and set Format On Save,
it will automatically format files when you save them.
ShellCheck
Just install the extension and it will display the results of static analysis in PROBLEMS
by ShellCheck as shown in the image. In the example in the image, the following two points are warned
- Use of glob or loop instead of
ls | grep
is recommended - The parameter expansion
$
is not necessary in arithmetic expression expansion
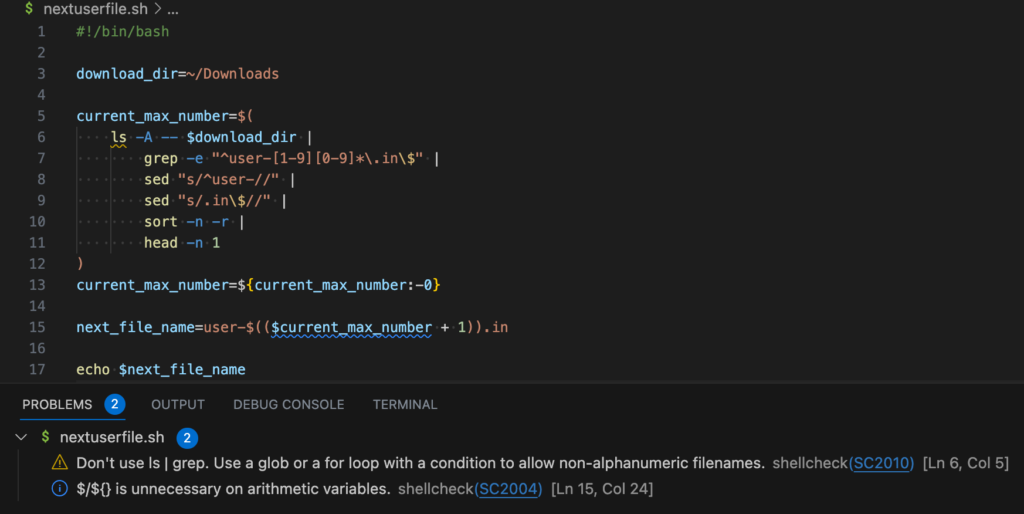
The page that can be opened from the link in the warning statement summarizes the reasons for the warnings and suggestions for correction. In addition, those that can be fixed mechanically can be fixed simply by selecting the Apply fix
that appears by hovering the mouse over the code being warned about.
Bash Debug
You can debug using the debugger by installing the extension, updating the Bash version to 4.0 or higher, and adding .vscode/launch.json
.
Bash version 4.0 or higher is required as a dependency of Bash Debug. In recent macOS, the default Shell is switched to Zsh, and the Bash version is initially 3.2, which is old. If you want to use the debugger with Zsh, you can use Zsh Debug instead of Bash Debug.
In .vscode/launch.json
you can configure the debug runtime settings. "terminalKind": "integrated"
is needed to provide input from the terminal if standard input is required for commands such as read
during shell execution. Also, if you need additional arguments for execution, you can add them to this file as "args": ["param1", "param2"]
.
Below is a brief description of how to use Bash Debug.
Breakpoints
Breakpoints can be set and released by clicking on the left side of the code.
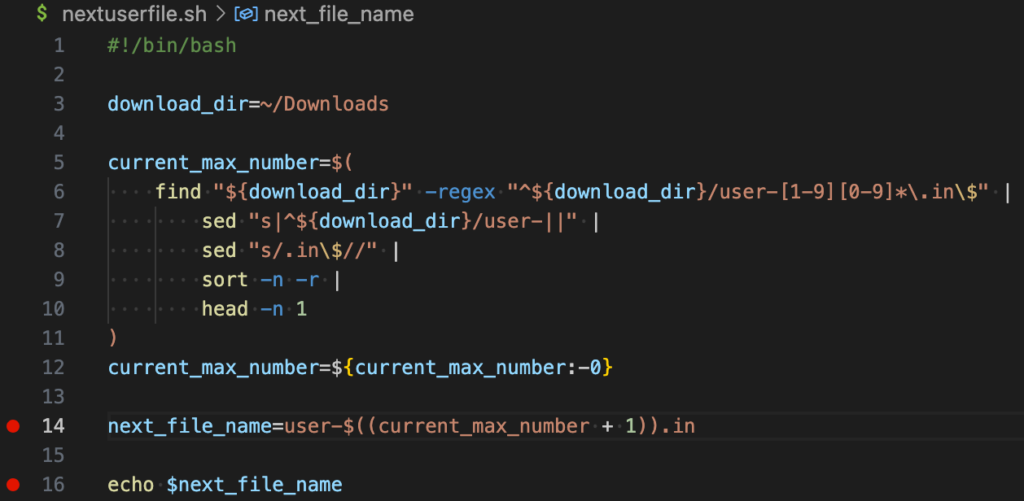
Start the debugger
F5
to start debugging. Execution stops at the breakpoint. At this time, if a parameter is entered in WATCH
, the value at that time will be displayed. You can also check the call stack of the function currently being called in CALLSTACK
.
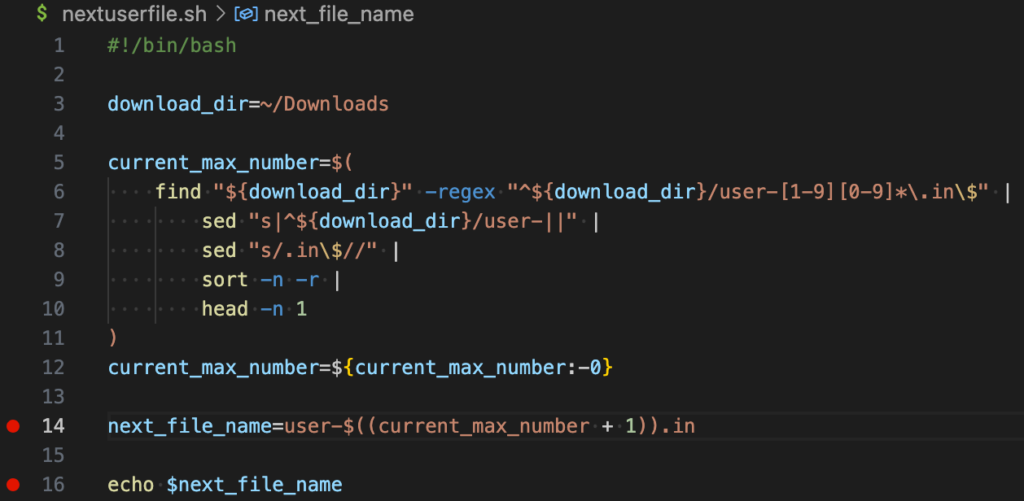
Impressions
This time, we used VSCode to set up a Shell development environment, mainly Bash. Since I have been writing Bash scripts almost exclusively in text files, development from now on will be easier. When development becomes easier, I feel like writing useful scripts more easily. Even if you haven’t written that many Shell scripts yet, I would recommend that you with a formality.
I also applied formatter and static analysis to the scripts I wrote in the past. The most common warnings in the static analysis were the lack of quoting in cases where quoting was required for parameter expansion, and the lack of the -r
option when calling the read
command. From now on, with the help of tools, I will be able to write better code than before without having to be particularly conscious of these things.